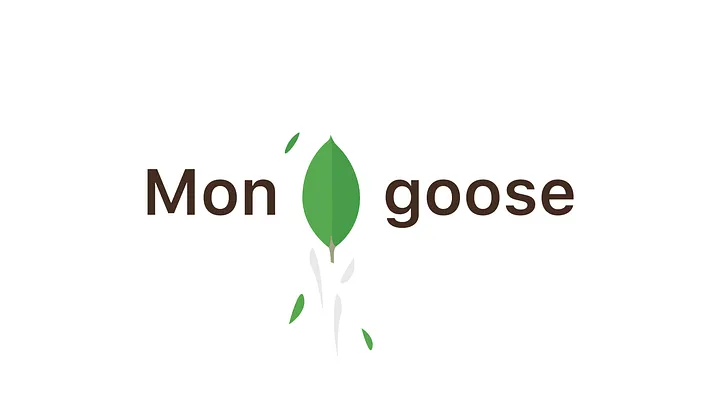
Efficiently Handling Large Datasets with Mongoose QueryCursor
When working with MongoDB and Mongoose, you may encounter scenarios where you need to handle large datasets efficiently. Loading an entire dataset into memory is not only impractical but can also lead to performance issues, such as high memory consumption or application crashes. Mongoose provides an elegant solution to this problem: the QueryCursor.
In this blog post, we’ll explore what a QueryCursor is, when to use it, and how to integrate it into your Node.js application for efficient data processing.
What is a QueryCursor?
A QueryCursor in Mongoose is a powerful tool that allows you to iterate over MongoDB query results one document at a time. Instead of fetching all documents into memory at once, it streams documents from the database, ensuring that only a small subset of data resides in memory at any given moment.
This makes QueryCursor particularly useful when dealing with large datasets or when performing batch processing.
Why Use a QueryCursor?
Here are some scenarios where a QueryCursor is ideal:
-
Memory Efficiency: Large datasets won’t overwhelm your application’s memory.
-
Batch Processing: Process data as it streams rather than waiting for the entire dataset to load.
-
Real-Time Applications: Stream data directly to a client or another service.
-
Controlled Iteration: Handle each document individually, making it easier to debug and monitor progress.
How to Use a QueryCursor
Using a QueryCursor in Mongoose is straightforward. Let’s walk through a basic example.
1. Setting up Mongoose
Ensure you have Mongoose installed and connected to a MongoDB instance:
const mongoose = require('mongoose');
mongoose.connect('mongodb://localhost:27017/mydatabase', {
useNewUrlParser: true,
useUnifiedTopology: true,
});
const UserSchema = new mongoose.Schema({
name: String,
age: Number,
});
const User = mongoose.model('User', UserSchema);
2. Creating a QueryCursor
To use a QueryCursor, call .cursor()
on a Mongoose query:
const cursor = User.find({ age: { $gte: 18 } }).cursor();
This creates a cursor for all users aged 18 and older.
3. Iterating Over the Cursor
You can iterate over the cursor using the for await...of
syntax, which works seamlessly with Node.js streams:
(async () => {
try {
for await (const user of cursor) {
console.log(user.name);
// Perform any desired processing on the user document
}
} catch (error) {
console.error('Error processing documents:', error);
} finally {
// Ensure the cursor is closed
await cursor.close();
}
})();
Alternatively, use the cursor.next()
method to manually fetch documents:
(async () => {
try {
let doc;
while ((doc = await cursor.next())) {
console.log(doc.name);
}
} catch (error) {
console.error('Error processing documents:', error);
} finally {
await cursor.close();
}
})();
QueryCursor with Transform Streams
You can also pipe a QueryCursor into a transform stream to process data on the fly. This is particularly useful when streaming data to a client or another service.
const { Transform } = require('stream');
const transformStream = new Transform({
objectMode: true,
transform(doc, encoding, callback) {
const transformed = {
...doc.toObject(),
processedAt: new Date(),
};
callback(null, transformed);
},
});
User.find({ age: { $gte: 18 } })
.cursor()
.pipe(transformStream)
.on('data', (data) => {
console.log('Transformed Data:', data);
})
.on('error', (error) => {
console.error('Stream Error:', error);
});
Best Practices for Using QueryCursor
To make the most of QueryCursor, follow these best practices:
- Close the Cursor: Always close the cursor after use to release database resources.
await cursor.close();
-
Handle Errors Gracefully: Wrap your code in try-catch blocks to handle errors during iteration.
-
Limit the Dataset: Use filters and projections to limit the amount of data being streamed, ensuring optimal performance.
const cursor = User.find({ age: { $gte: 18 } }).select('name age').cursor();
- Monitor Performance: Test the performance of your queries with large datasets and optimize your MongoDB indexes as needed.
Conclusion
Mongoose’s QueryCursor is an essential tool for developers working with large datasets. By streaming data one document at a time, it ensures efficient memory usage and enables powerful batch processing workflows. Whether you’re building data pipelines or real-time applications, QueryCursor helps you handle data effectively without sacrificing performance.
Start using QueryCursor in your projects today and unlock the power of efficient data handling with Mongoose!
Happy Coding!!!